Another use for AnimationCurves in Unity3D is to adjust the speed of things without changing variables, or adding complicated curves. The variable we want returned is the Y-Point (a float from 0.0 to 4.0), based on time (from 0.0 to 1.0, going left to right).
As time gets closer to 1.0, the Y-point decreases from 4.0 to 1.0.
1
| powerbarReverse.fillAmount += Time.deltaTime / powerCurveStart.Evaluate (powerbarReverse.fillAmount); |
powerbarReverse is a UI Image whose image type is filled. As you change the fill amount from 0.0 to 1.0, the image fills in, in the Fill Origin type specified in the inspector.
powerCurveStart is the Animation curve below. As time goes on, the image will fill faster, as Time.delta time gets divided by a smaller number.
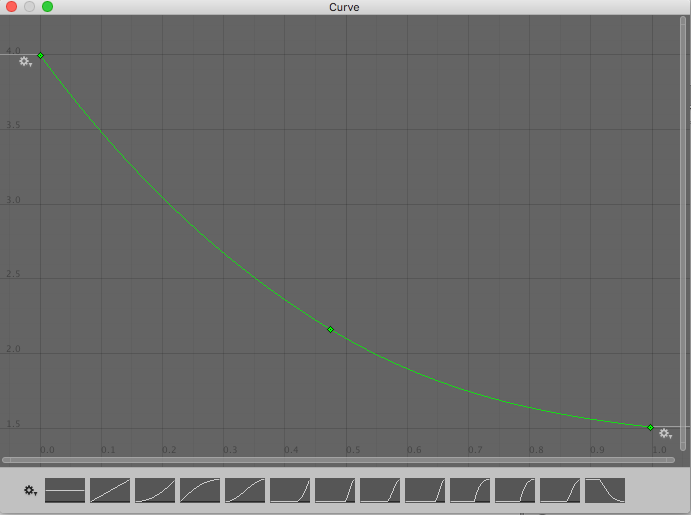
AnimationCurve used when the Power Bar is moving forward (increasing).
1
| powerbarReverse.fillAmount -= Time.deltaTime / powerCurveEnd.Evaluate (powerbarReverse.fillAmount); |
powerCurveEnd is the Animation curve below. It will start at 1.0, and the image will unfill as time goes on. It starts fast, and slows down. When it reaches 0.33 is the speed will stay constant. The speed stays slow and constant at this point so players can decide if they want to add spin to the bowling ball to make it hook left or right.
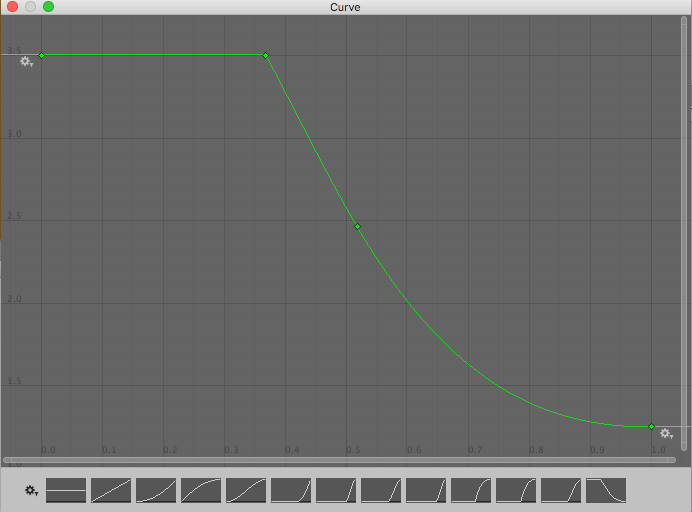
AnimationCurve used when the Power Bar is reversing (decreasing).
To add spin or hook the ball, the player would stop the power bar on either side of the “bump” in the left side of the power bar meter. If the player stops it where shown below, the ball will have no spin and roll perfectly straight down the alley.
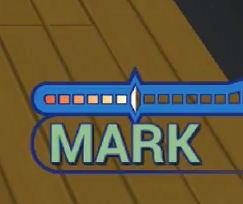
Sample code is below. A UI.Button calls the SwingClub() routine, which starts, reverses and stops the power bar with each press.
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40
| <span style="font-family: Menlo;"><span style="color: #009695;"><span style="font-family: Menlo;"><span style="color: #009695;">private</span><span style="color: #333333;"> </span><span style="color: #009695;">int</span><span style="color: #333333;"> </span><span style="color: #333333;">pbDirection</span><span style="color: #333333;"> </span><span style="color: #333333;">=</span><span style="color: #333333;"> </span><span style="color: #f57d00;">0</span><span style="color: #333333;">;</span></span>
public</span><span style="color: #333333;"> </span><span style="color: #3364a4;">Image</span><span style="color: #333333;"> </span><span style="color: #333333;">powerbarForward</span><span style="color: #333333;">;</span>
<span style="color: #009695;">public</span><span style="color: #333333;"> </span><span style="color: #3364a4;">Image</span><span style="color: #333333;"> </span><span style="color: #333333;">powerbarReverse</span><span style="color: #333333;">;</span></span>
<span style="font-family: Menlo;"><span style="color: #009695;">public</span><span style="color: #333333;"> </span><span style="color: #3364a4;">AnimationCurve</span><span style="color: #333333;"> </span><span style="color: #333333;">powerCurveEnd</span><span style="color: #333333;">;</span>
<span style="color: #009695;">public</span><span style="color: #333333;"> </span><span style="color: #3364a4;">AnimationCurve</span><span style="color: #333333;"> </span><span style="color: #333333;">powerCurveStart</span><span style="color: #333333;">;</span></span>
<span style="font-family: Menlo;"><span style="color: #009695;">public</span><span style="color: #333333;"> </span><span style="color: #009695;">enum</span><span style="color: #333333;"> </span><span style="color: #3364a4;">swingType</span><span style="color: #333333;"> </span><span style="color: #333333;">{</span><span style="color: #333333;"> swingInactive</span><span style="color: #333333;">,</span><span style="color: #333333;"> startSwing</span><span style="color: #333333;">,</span><span style="color: #333333;"> stopSwing</span><span style="color: #333333;">,</span><span style="color: #333333;"> endSwing</span><span style="color: #333333;">,</span><span style="color: #333333;"> BallThrown </span><span style="color: #333333;">}</span><span style="color: #333333;">;</span>
<span style="color: #009695;">public</span><span style="color: #333333;"> </span><span style="color: #3364a4;">swingType</span><span style="color: #333333;"> </span><span style="color: #333333;">actionstate</span><span style="color: #333333;">;
<span style="font-family: Menlo;"><span style="color: #009695;">public</span><span style="color: #333333;"> </span><span style="color: #009695;">void</span><span style="color: #333333;"> </span><span style="color: #333333;">SwingClub</span><span style="color: #333333;">()</span>
<span style="color: #333333;">{</span>
<span style="color: #333333;"> </span><span style="color: #009695;">switch</span><span style="color: #333333;">(</span><span style="color: #333333;">actionstate</span><span style="color: #333333;">)</span>
<span style="color: #333333;"> </span><span style="color: #333333;">{</span>
<span style="color: #333333;"> </span><span style="color: #009695;">case</span><span style="color: #333333;"> </span><span style="color: #3364a4;">swingType</span><span style="color: #333333;">.</span><span style="color: #333333;">swingInactive</span><span style="color: #333333;">:</span><span style="color: #333333;"> </span><span style="color: #333333;">StartSwing</span><span style="color: #333333;">()</span><span style="color: #333333;">;</span><span style="color: #333333;"> </span><span style="color: #009695;">break</span><span style="color: #333333;">;</span>
<span style="color: #333333;"> </span><span style="color: #009695;">case</span><span style="color: #333333;"> </span><span style="color: #3364a4;">swingType</span><span style="color: #333333;">.</span><span style="color: #333333;">startSwing</span><span style="color: #333333;">:</span><span style="color: #333333;"> </span><span style="color: #333333;">StopSwing</span><span style="color: #333333;">()</span><span style="color: #333333;">;</span><span style="color: #333333;"> </span><span style="color: #009695;">break</span><span style="color: #333333;">;</span><span style="color: #333333;"> </span>
<span style="color: #333333;"> </span><span style="color: #009695;">case</span><span style="color: #333333;"> </span><span style="color: #3364a4;">swingType</span><span style="color: #333333;">.</span><span style="color: #333333;">stopSwing</span><span style="color: #333333;">:</span><span style="color: #333333;"> </span><span style="color: #333333;">EndSwing</span><span style="color: #333333;">()</span><span style="color: #333333;">;</span><span style="color: #009695;">break</span><span style="color: #333333;">;</span><span style="color: #333333;"> </span>
<span style="color: #333333;"> </span><span style="color: #333333;">}</span>
<span style="color: #333333;">}</span>
<span style="color: #009695;">void</span><span style="color: #333333;"> </span><span style="color: #333333;">StartSwing</span><span style="color: #333333;">()</span>
<span style="color: #333333;">{</span>
<span style="color: #333333;"> </span><span style="color: #009695;">if</span><span style="color: #333333;">(</span><span style="color: #333333;">playerReadyButtonActive</span><span style="color: #333333;">)</span><span style="color: #333333;"> </span><span style="color: #333333;">{</span><span style="color: #333333;"> </span><span style="color: #009695;">return</span><span style="color: #333333;">;</span><span style="color: #333333;"> </span><span style="color: #333333;">}</span>
<span style="color: #333333;"> </span><span style="color: #333333;">actionstate</span><span style="color: #333333;"> </span><span style="color: #333333;">=</span><span style="color: #333333;"> </span><span style="color: #3364a4;">swingType</span><span style="color: #333333;">.</span><span style="color: #333333;">startSwing</span><span style="color: #333333;">;</span>
<span style="color: #333333;"> </span><span style="color: #333333;">pbDirection</span><span style="color: #333333;"> </span><span style="color: #333333;">=</span><span style="color: #333333;"> </span><span style="color: #f57d00;">1</span><span style="color: #333333;">;</span>
<span style="color: #333333;"> </span><span style="color: #333333;">StartCoroutine</span><span style="color: #333333;"> </span><span style="color: #333333;">(</span><span style="color: #333333;">MovePowerBar</span><span style="color: #333333;">())</span><span style="color: #333333;">;</span>
<span style="color: #333333;">}</span>
<span style="color: #009695;">void</span><span style="color: #333333;"> </span><span style="color: #333333;">StopSwing</span><span style="color: #333333;">()</span>
<span style="color: #333333;">{</span>
<span style="color: #333333;"> </span><span style="color: #333333;">powerbarForward</span><span style="color: #333333;">.</span><span style="color: #333333;">fillAmount</span><span style="color: #333333;"> </span><span style="color: #333333;">=</span><span style="color: #333333;"> </span><span style="color: #333333;">powerbarReverse</span><span style="color: #333333;">.</span><span style="color: #333333;">fillAmount</span><span style="color: #333333;">;</span>
<span style="color: #333333;"> </span><span style="color: #333333;">pbDirection</span><span style="color: #333333;"> </span><span style="color: #333333;">=</span><span style="color: #333333;"> </span><span style="color: #333333;">-</span><span style="color: #f57d00;">1</span><span style="color: #333333;">;</span>
<span style="color: #333333;"> </span><span style="color: #333333;">actionstate</span><span style="color: #333333;"> </span><span style="color: #333333;">=</span><span style="color: #333333;"> </span><span style="color: #3364a4;">swingType</span><span style="color: #333333;">.</span><span style="color: #333333;">stopSwing</span><span style="color: #333333;">;</span>
<span style="color: #333333;">}</span>
<span style="color: #009695;">void</span><span style="color: #333333;"> </span><span style="color: #333333;">EndSwing</span><span style="color: #333333;">()
</span><span style="color: #333333;">{</span>
<span style="color: #333333;"> </span><span style="color: #333333;">pbDirection</span><span style="color: #333333;"> </span><span style="color: #333333;">=</span><span style="color: #333333;"> </span><span style="color: #f57d00;">0</span><span style="color: #333333;">;</span>
<span style="color: #333333;"> </span><span style="color: #333333;">actionstate</span><span style="color: #333333;"> </span><span style="color: #333333;">=</span><span style="color: #333333;"> </span><span style="color: #3364a4;">swingType</span><span style="color: #333333;">.</span><span style="color: #333333;">endSwing</span><span style="color: #333333;">;</span>
<span style="color: #333333;">}</span>
</span> </span></span>
IEnumerator MovePowerBar () |
1
| while (pbDirection != 0) |
1
| powerbarReverse.fillAmount += Time.deltaTime / powerCurveStart.Evaluate (powerbarReverse.fillAmount); |
1
| if (powerbarReverse.fillAmount >= 1.0f) |
1
| powerbarForward.fillAmount = 1f; |
1
| actionstate = swingType.stopSwing; |
1
| powerbarReverse.fillAmount -= Time.deltaTime / powerCurveEnd.Evaluate (powerbarReverse.fillAmount); |
1
| if (powerbarReverse.fillAmount <= 0f) |
1
| actionstate = swingType.endSwing; |